Physical Simulations
Exponential Curves
Now let's look at the exponential curve, implemented in JavaScript as Math.exp(). This function takes whatever parameter you pass it and raises the constant "e" (2.718...) to that power. The wave that you get from this function really depends on what parameter you pass it. If we were to use time as the parameter, for example, our function would give us the value 1 where time is zero and begin increasing very rapidly (exponentially in fact) as time becomes greater than zero. Take a look at the graph of Math.exp(time).
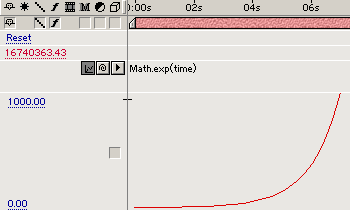
exponential curve
Notice that by around 7 seconds the value is already around 1000 and increasing rapidly.
Exponential Decay
The exponential curve certainly has its uses, but we will more often be interested in the flavor that decreases exponentially rather than increases. This will be very useful for simulating things such as bouncing balls, springs, etc. - or anything that gradually slows down. There are two ways to generate this decaying curve and they give identical results. The first way is just to make the parameter to Math.exp() negative. For example, here's the graph of Math.exp(-time).
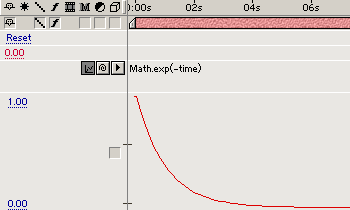
decaying exponential curve
You can see that at time zero the value is 1 and as time increases, the value gradually approaches zero.
The other variation of the decaying exponential curve is obtained simply by supplying the Math.exp() function with a positive parameter and dividing 1 by the result. That correctly implies that 1/Math.exp(time) is the same as Math.exp(-time).
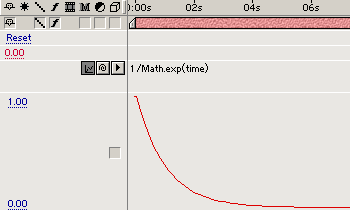
alternate decaying exponential curve
As you can see, the curves are identical.
Double Trouble
Before we move on to some practical applications of these math functions we need to take a look at the extremely useful combination of Math.sin() and Math.exp(). When we combine the sine wave with the decaying exponential curve, we get a decaying oscillation, which is very useful for simulating springs, pendulums, etc. Here's the graph of Math.sin(6*time)/Math.exp(time). From our discussion above, we know that Math.sin(6*time)*Math.exp(-time) would give us the same result.
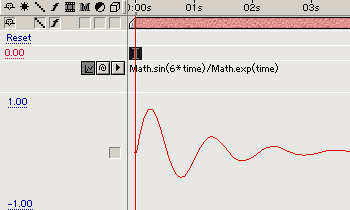
decaying sine wave
You'll notice that what we get is a sine wave that quickly decays towards 0. (In case you're wondering where the 6 came from, it just speeds up the frequency of the sine wave to better demonstrate the decaying effect (we'll cover this "speeding up" of the sine wave in more detail shortly.)
Setting Up Parameters
What we need to do now is set up some parameters to let us easily control the frequency and amplitude of the sine and cosine waves, and the rate of decay of the decaying exponential curve. The amplitude is easy - we'll just have a variable called "amplitude" that we multiply by the Math.sin() function. If we set "amplitude" equal to 100, for example, our sine wave will range from -100 to +100 instead of -1 to +1.
Frequency is also easy. Using the information we discussed previously about Math.sin() and Math.cos() wanting their parameters in radians, we just create a variable called "freq" and set it to the number of oscillations per second that we want. Then all we have to do is use Math.sin(freq*time*2*Math.PI) to generate the oscillation.
Finally, let's look at what it takes to make our exponential decay faster or slower. It turns out that all we need is a parameter that we'll call "decay" that we multiply the time by in the Math.exp() function. If "decay" is zero, there will be no decay - the sine wave will just keep going. If "decay" is 1, the decay rate will be like in the examples above. Between zero and 1, the decay will be slower. Greater than 1, faster.
![]() |